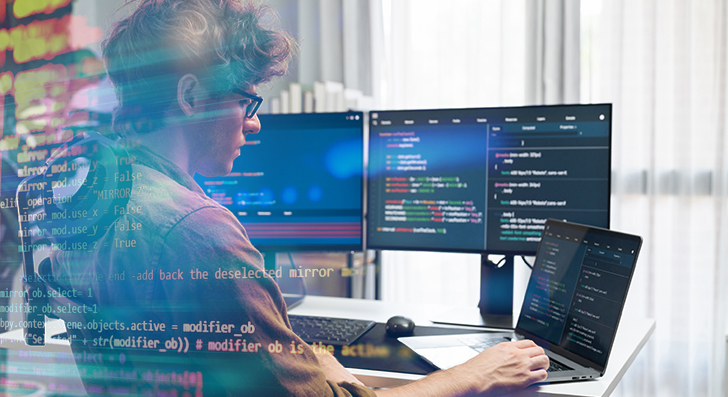
Scalability usually means your software can cope with expansion—a lot more consumers, much more info, and even more site visitors—with out breaking. As a developer, setting up with scalability in mind will save time and tension later. Below’s a clear and practical guideline to assist you to start off by Gustavo Woltmann.
Layout for Scalability from the Start
Scalability isn't a little something you bolt on later on—it should be section of the plan from the start. Numerous applications are unsuccessful once they mature rapidly simply because the first design and style can’t handle the extra load. As a developer, you must Believe early regarding how your technique will behave under pressure.
Start out by creating your architecture to generally be versatile. Stay clear of monolithic codebases where almost everything is tightly related. As an alternative, use modular design or microservices. These designs crack your app into more compact, independent components. Each individual module or support can scale By itself without influencing The complete program.
Also, contemplate your database from day 1. Will it need to manage a million end users or simply 100? Pick the proper style—relational or NoSQL—dependant on how your knowledge will grow. Approach for sharding, indexing, and backups early, Even though you don’t will need them however.
One more vital level is to stay away from hardcoding assumptions. Don’t write code that only functions beneath present-day circumstances. Think of what would transpire In the event your person base doubled tomorrow. Would your app crash? Would the database slow down?
Use layout styles that assistance scaling, like concept queues or celebration-pushed techniques. These assistance your application tackle much more requests with no having overloaded.
If you build with scalability in mind, you're not just getting ready for achievement—you're lowering long run headaches. A effectively-planned technique is less complicated to take care of, adapt, and grow. It’s much better to prepare early than to rebuild later.
Use the correct Database
Selecting the appropriate database can be a essential Component of building scalable programs. Not all databases are built exactly the same, and utilizing the Completely wrong you can sluggish you down or perhaps cause failures as your app grows.
Get started by being familiar with your data. Can it be remarkably structured, like rows in a very table? If yes, a relational databases like PostgreSQL or MySQL is an efficient match. They are potent with interactions, transactions, and regularity. They also assistance scaling procedures like read through replicas, indexing, and partitioning to handle additional site visitors and details.
When your data is a lot more versatile—like person activity logs, products catalogs, or paperwork—consider a NoSQL selection like MongoDB, Cassandra, or DynamoDB. NoSQL databases are much better at managing big volumes of unstructured or semi-structured details and will scale horizontally much more quickly.
Also, look at your browse and write patterns. Have you been performing a great deal of reads with fewer writes? Use caching and read replicas. Will you be handling a weighty generate load? Consider databases which will deal with substantial create throughput, as well as occasion-based mostly facts storage systems like Apache Kafka (for short-term facts streams).
It’s also smart to Believe forward. You might not need State-of-the-art scaling features now, but choosing a database that supports them implies you gained’t have to have to modify later.
Use indexing to speed up queries. Stay away from unneeded joins. Normalize or denormalize your information according to your entry styles. And always keep track of database efficiency while you improve.
Briefly, the ideal database relies on your application’s composition, velocity requires, And exactly how you hope it to grow. Take time to pick wisely—it’ll save a lot of problems later on.
Enhance Code and Queries
Rapid code is vital to scalability. As your app grows, every single tiny delay adds up. Improperly written code or unoptimized queries can decelerate functionality and overload your technique. That’s why it’s crucial that you Create efficient logic from the start.
Get started by creating cleanse, simple code. Steer clear of repeating logic and remove nearly anything pointless. Don’t choose the most complex Alternative if a straightforward just one performs. Maintain your functions shorter, centered, and simple to check. Use profiling applications to uncover bottlenecks—destinations wherever your code usually takes far too extensive to operate or works by using far too much memory.
Future, check out your database queries. These generally sluggish factors down greater than the code itself. Ensure that Every single question only asks for the information you actually have to have. Keep away from Pick *, which fetches every thing, and alternatively choose precise fields. Use indexes to speed up lookups. And prevent performing a lot of joins, Particularly throughout large tables.
In case you notice the exact same information becoming asked for repeatedly, use caching. Retail store the outcomes quickly using equipment like Redis or Memcached so that you don’t really have to repeat expensive operations.
Also, batch your databases functions whenever you can. Instead of updating a row one by one, update them in teams. This cuts down on overhead and makes your app a lot more successful.
Make sure to check with massive datasets. Code and queries that do the job fine with 100 information could possibly crash once they have to deal with 1 million.
To put it briefly, scalable applications are fast apps. Maintain your code restricted, your queries lean, and use caching when wanted. These ways help your application remain easy and responsive, at the same time as the load improves.
Leverage Load Balancing and Caching
As your application grows, it has to handle a lot more people plus more traffic. If everything goes via a single server, it's going to speedily become a bottleneck. That’s where by load balancing and caching are available. Both of these instruments enable maintain your app quickly, stable, and scalable.
Load balancing spreads incoming visitors across multiple servers. Instead of one server doing all the perform, the load balancer routes consumers to various servers according to availability. This means no one server will get overloaded. If a single server goes down, the load balancer can deliver traffic to the Many others. Instruments like Nginx, HAProxy, or cloud-based mostly remedies from AWS and Google Cloud make this simple to set up.
Caching is about storing info temporarily so it might be reused speedily. When customers ask for the exact same facts once again—like a product web site or possibly a profile—you don’t have to fetch it from the databases each time. You could serve it with the cache.
There are 2 common sorts of caching:
1. Server-facet caching (like Redis or Memcached) retailers details in memory for rapidly access.
two. Client-aspect caching (like browser caching or CDN caching) shops static documents close to the user.
Caching lessens database load, increases speed, and would make your app additional efficient.
Use caching for things which don’t change typically. And always be sure your cache is updated when knowledge does change.
In brief, load balancing and caching are uncomplicated but powerful equipment. Alongside Gustavo Woltmann blog one another, they help your app tackle much more end users, continue to be quickly, and Get better from issues. If you intend to mature, you'll need equally.
Use Cloud and Container Resources
To develop scalable purposes, you'll need resources that allow your application mature effortlessly. That’s in which cloud platforms and containers can be found in. They offer you flexibility, decrease setup time, and make scaling Considerably smoother.
Cloud platforms like Amazon Website Services (AWS), Google Cloud Platform (GCP), and Microsoft Azure Enable you to hire servers and services as you'll need them. You don’t must get components or guess long run potential. When traffic raises, you'll be able to incorporate far more methods with just a couple clicks or mechanically working with vehicle-scaling. When visitors drops, you are able to scale down to save money.
These platforms also provide solutions like managed databases, storage, load balancing, and safety equipment. You'll be able to give attention to constructing your app as opposed to handling infrastructure.
Containers are Yet another important Instrument. A container packages your application and anything it needs to operate—code, libraries, configurations—into just one device. This causes it to be uncomplicated to maneuver your app in between environments, from your notebook into the cloud, without the need of surprises. Docker is the preferred Resource for this.
Whenever your app uses various containers, equipment like Kubernetes enable you to handle them. Kubernetes handles deployment, scaling, and recovery. If a person aspect of the app crashes, it restarts it automatically.
Containers also enable it to be very easy to separate aspects of your app into services. You may update or scale elements independently, which is perfect for overall performance and trustworthiness.
In a nutshell, employing cloud and container tools suggests you are able to scale speedy, deploy very easily, and Get better speedily when problems come about. If you would like your application to develop without the need of limitations, get started making use of these applications early. They preserve time, cut down chance, and assist you to stay focused on setting up, not fixing.
Monitor Every little thing
For those who don’t check your software, you received’t know when issues go Erroneous. Monitoring aids the thing is how your application is carrying out, place challenges early, and make better choices as your application grows. It’s a vital Component of setting up scalable methods.
Commence by monitoring essential metrics like CPU usage, memory, disk Area, and response time. These inform you how your servers and products and services are doing. Resources like Prometheus, Grafana, Datadog, or New Relic may help you obtain and visualize this data.
Don’t just check your servers—keep an eye on your application much too. Keep an eye on how long it will take for consumers to load internet pages, how frequently errors happen, and exactly where they take place. Logging equipment like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly may help you see what’s occurring within your code.
Put in place alerts for critical complications. For example, In case your response time goes over a limit or perhaps a services goes down, you need to get notified instantly. This helps you fix issues fast, usually just before consumers even recognize.
Monitoring is also practical any time you make improvements. In case you deploy a fresh characteristic and see a spike in faults or slowdowns, you may roll it back again prior to it causes serious problems.
As your app grows, visitors and data raise. With no monitoring, you’ll pass up signs of problems till it’s much too late. But with the appropriate resources set up, you keep in control.
Briefly, monitoring can help you keep your application reputable and scalable. It’s not just about recognizing failures—it’s about comprehending your procedure and making certain it works properly, even under pressure.
Closing Thoughts
Scalability isn’t only for big corporations. Even little applications require a solid Basis. By creating thoroughly, optimizing properly, and utilizing the correct instruments, it is possible to Establish apps that improve efficiently devoid of breaking stressed. Begin tiny, Assume large, and Create good.